Developer Guide
- Setting up, getting started
- Design
- Implementation
- Documentation, logging, testing, configuration, dev-ops
-
Appendix: Requirements
- Product scope
- User stories
- Use cases
- UC01: Enters a command:
- UC02: Enters Help Command:
- UC03: Add a patient
- UC04: Clear Patient Records
- UC05: Delete a patient
- UC06: Edit a patient
- UC07: Find patients by search fields
- UC08: List all patients
- UC09: Add a doctor
- UC10: Clear Doctor Records
- UC11: Delete a doctor
- UC12: Edit a doctor
- UC13: Find doctors by search fields
- UC14: List all doctors
- UC15: Add an appointment
- UC16: Clear Appointment Schedule
- UC17: Delete an appointment
- UC18: Edit an appointment
- UC19: Find appointments by search fields
- UC20: List all appointments
- Non-Functional Requirements
- Glossary
-
Appendix: Instructions for manual testing
- Launch and shutdown
- Adding a patient
- Adding a doctor
- Adding an appointment
- Clearing patient records (or doctor records)
- Clearing appointment schedule
- Deleting a patient (or doctor)
- Editing a patient
- Editing a doctor
- Edit an appointment
- Find patients by search fields
- Listing patient records (or doctor records, or appointment schedule)
- Reading data from storage
- Appendix: Effort
Setting up, getting started
Refer to the guide Setting up and getting started.
Design
Architecture
The Architecture Diagram given above explains the high-level design of the App. Given below is a quick overview of each component.
Main
has two classes called Main
and MainApp
. It is responsible for,
- At app launch: Initializes the components in the correct sequence, and connects them up with each other.
- At shut down: Shuts down the components and invokes cleanup methods where necessary.
Commons
represents a collection of classes used by multiple other components.
The rest of the App consists of four components.
-
UI
: The UI of the App. -
Logic
: The command executor. -
Model
: Holds the data of the App in memory. -
Storage
: Reads data from, and writes data to, the hard disk.
Each of the four components,
- defines its API in an
interface
with the same name as the Component. - exposes its functionality using a concrete
{Component Name}Manager
class (which implements the corresponding APIinterface
mentioned in the previous point.
For example, the Logic
component (see the class diagram given below) defines its API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class which implements the Logic
interface.
How the architecture components interact with each other
The Sequence Diagram below shows how the components interact with each other for the scenario where the user issues the command delete-patient 1
.
The component specifics are elaborated in the following sections.
The Appointment Diagram given above explains the interactions between the fundamental classes of the App: Appointment
, Patient
and Doctor
.
-
Patient
andDoctor
are extensions of thePerson
abstract class which defines the criterion for duplicate identities. -
PatientMap
andDoctorMap
maintain unique records of patients and doctors respectively via the assignment ofUUID
. -
Appointment
stores theUUID
and accessesPatient
andDoctor
data throughDoctorMap
andPatientMap
respectively. - Conflicts between appointments are then defined by duplicate persons bounded by overlapping
Timeslot
.
This interaction between the 3 classes maintain the integrity of the App’s appointment schedule, patient records and doctor records.
UI component
API :
Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
,PatientListPanel
, DoctorListPanel
, AppointmentListPanel
, StatusBarFooter
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
- Executes user commands using the
Logic
component. - Listens for changes to
Model
data so that the UI can be updated with the modified data.
Logic component
API :
Logic.java
-
Logic
uses theUserInputParser
class to parse the user command. - This results in a
Command
object which is executed by theLogicManager
. - The command execution can affect the
Model
andStorage
(e.g. adding a person). - The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
. - In addition, the
CommandResult
object can also instruct theUi
to perform certain actions, such as displaying help to the user.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("delete-patient 1")
API call.

DeleteCommandParser
should end at the destroy marker (X) but due to a limitation of PlantUML, the lifeline reaches the end of diagram.
Model component
API : Model.java
The Model
,
- stores a
UserPref
object that represents the user’s preferences. - stores the address book data and appointment schedule data.
- exposes unmodifiable
ObservableList<Person>
andObservableList<Appointment>
that can be ‘observed’ e.g. the UI can be bound to these lists so that the UI automatically updates when the data in these lists change. - does not depend on any of the other three components.
The Person
,
- stores a
Person
class, which theDoctor
class andPatient
class inherit from.
The Appointment
,
- stores a
Appointment
class, which accessPatient
object andDoctor
object via thePatientMap
class andDoctorMap
class.

Tag
list in the AddressBook
, which Person
references. This allows AddressBook
to only require one Tag
object per unique Tag
, instead of each Person
needing their own Tag
object. Similar design can be applied to the Appointment model.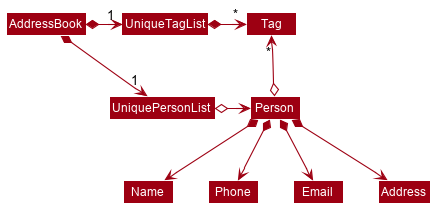
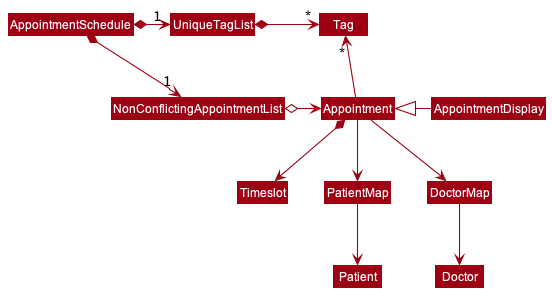
Storage component
API : Storage.java
The Storage
component,
- can save
UserPref
objects in json format and read it back. - can save the
PatientRecords
data in json format and read it back. - can save the
DoctorRecords
data in json format and read it back. - can save the
AppointmentSchedule
data in json format and read it back.
Common classes
Classes used by multiple components are in the seedu.addressbook.commons
package.
Implementation
This section describes some noteworthy details on how certain features are implemented.
Documentation, logging, testing, configuration, dev-ops
Appendix: Requirements
Product scope
Target user profile: App-Ointment is intended for Receptionists of Medical Clinics who help schedule appointments, and maintain patient records and accounts.
- has a need to manage a significant number of scheduled appointments
- prefer desktop apps over other types
- can type fast
- prefers typing to mouse interactions
- is reasonably comfortable using CLI apps
Value proposition
- Allows users to track and reschedule appointments for a clinic, reducing no-shows.
- Allow users to verify the patient on arriving at the clinic for the appointment.
- No cross clinic support for clinics within a health group.
- No support for users who want to view their own appointments.
User stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
* * * |
new user | see usage instructions | refer to instructions when I forget how to use the App |
* * * |
user | add a new patient | |
* * * |
user | add a new doctor | |
* * * |
user | add a new appointment | |
* * * |
user | delete a patient | remove patients who on longer have appointments |
* * * |
user | delete a doctor | remove doctors who on longer work for the clinic |
* * * |
user | delete an appointment | remove appointments that have expired or on behalf of the patient |
* * * |
user | edit a patient by specific fields | update the patient information without having to delete and add a new patient |
* * * |
user | edit a doctor by specific fields | update the doctor information without having to delete and add a new doctor |
* * * |
user | edit an appointment by specific fields | update the appointment information without having to delete and add a new appointment |
* * * |
user | find a patient by specific fields | locate details of relevant patients without having to go through the entire list |
* * * |
user | find a doctor by specific fields | locate details of relevant doctors without having to go through the entire list |
* * * |
user | find an appointment by specific fields | locate details of relevant appointments without having to go through the entire list |
* * * |
user | list all patients | see all the patients or reset the patient filters |
* * * |
user | list all doctors | see all the doctors or reset the doctors filters |
* * * |
user | list all appointments | see all the appointments or reset the appointment filters |
* * |
user | clear the patient records | clear the patient records without having to delete patients one by one |
* * |
user | clear the doctor records | clear the doctor records without having to delete doctors one by one |
* * |
user | clear the appointment schedule | clear the appointment schedule without having to delete appointments one by one |
* * |
user | look up previous records of a patient | fill in missing information where omitted by the patient |
* * |
user | look up previous records of an appointment | fill in missing information where omitted by the appointment |
* * |
user | look up previous records of a doctor | fill in missing information where omitted by the doctor |
* |
user with many appointments in the schedule | be reminded of overdue appointments | take the appropriate action to resolve the issues |
* |
user with many appointments in the schedule | tag appointments with urgency categories | more urgent appointments can take priority |
* |
user with many appointments in the schedule | sort appointments by specific fields | locate a category of appointments easily |
* |
user with many appointments in the schedule | automatically recommended available timings and doctors for new appointments | create appointments without manually checking availability in the schedule |
Use cases
(For all use cases below, the System is the App-Ointment
and the Actor is the User
, unless specified otherwise)
UC01: Enters a command:
Guarantees:
- Command entered will have a valid command word, with all corresponding required subcommands.
MSS
- User enters a command.
- App-Ointment attempts to perform the corresponding command.
Use case ends.
Extensions
-
1a. App-Ointment detects an invalid command word from the user.
-
1a1. App-Ointment prompts user that the command is invalid, and suggests the closest known command, or “unknown command”, if all known commands are too dissimilar.
Use case resumes from Step 1.
-
1a1. App-Ointment prompts user that the command is invalid, and suggests the closest known command, or “unknown command”, if all known commands are too dissimilar.
-
2a. App-Ointment detects that required subcommands are not specified.
- 2a1. App-Ointment prompts user that the command is invalid, i.e. The subcommands do not match the command word. Use case resumes from Step 1.
UC02: Enters Help Command:
MSS
- User enters the
help
command - App-Ointment shows the Help Window.
Use case ends.
UC03: Add a patient
MSS
- User enters the
add-patient
command and corresponding subcommands. - App-Ointment adds a new patient to the patient records.
Extensions
-
1a. App-Ointment detects an invalid subcommand format.
-
1a1. App-Ointment prompts user that syntax is incorrect and displays the expected format.
Steps 1a1 is repeated until the subcommand entered is correct/free from errors. Use case resumes from step 2.
-
1a1. App-Ointment prompts user that syntax is incorrect and displays the expected format.
-
1b. App-Ointment detects that a patient with the same name exists in the patient records.
-
1b1. App-Ointment warns user about the duplicate patient.
-
1b2. App-Ointment suggest user to update patient information through an
edit-patient
command instead.
Use case ends.
-
1b1. App-Ointment warns user about the duplicate patient.
UC04: Clear Patient Records
MSS
- User enters the
clear-patient
command. - App-Ointment clears the patient records.
Use case ends.
Extensions
-
1a App-Ointment detects at there is at least 1 appointment in the Appointment Schedule.
-
1a1 App-Ointment prompts user that there are appointments in the appointment schedule, and as such, patient records cannot be cleared, and prompts user to execute the
clear-appt
command first instead.
-
1a1 App-Ointment prompts user that there are appointments in the appointment schedule, and as such, patient records cannot be cleared, and prompts user to execute the
UC05: Delete a patient
MSS
- User enters the
delete-patient
command and corresponding subcommands. - App-Ointment removes the patient from the patient records.
Extensions
-
1a. App-Ointment detects an invalid subcommand format.
-
1a1. App-Ointment prompts user that syntax is incorrect and displays the expected format.
Steps 1a1 is repeated until the subcommand entered is correct/free from errors. Use case resumes from step 2.
-
1a1. App-Ointment prompts user that syntax is incorrect and displays the expected format.
-
1b. The currently displayed list of patients is empty.
-
1b1. App-Ointment prompts user that there are no patients in the current display.
Use case ends.
-
1b1. App-Ointment prompts user that there are no patients in the current display.
-
2a. The index out of the bounds of the displayed list of patients.
-
2a1. App-Ointment warns user that the index is out of bounds and displays the bounds of the displayed list of patients.
Steps 2a1 is repeated until the index entered is correct/free from errors. Use case resumes from step 2.
-
2a1. App-Ointment warns user that the index is out of bounds and displays the bounds of the displayed list of patients.
-
2b. App-Ointment detects at least 1 appointment associated with the patient identified by the index number used in the displayed list of patients.
-
2b1. App-Ointment warns user about the associated appointments, prompts user to use force delete and displays the expected subcommand format.
Use case ends.
-
2b1. App-Ointment warns user about the associated appointments, prompts user to use force delete and displays the expected subcommand format.
UC06: Edit a patient
MSS
- User enters the
edit-patient
command and corresponding subcommands. - App-Ointment changes the details of the patient.
Extensions
-
1a. App-Ointment detects an invalid subcommand format.
-
1a1. App-Ointment prompts user that syntax is incorrect and displays the expected format.
Steps 1a1 is repeated until the subcommand entered is correct/free from errors. Use case resumes from step 2.
-
1a1. App-Ointment prompts user that syntax is incorrect and displays the expected format.
-
1b. The currently displayed list of patients is empty.
-
1b1. App-Ointment prompts user that there are no patients in the current display.
Use case ends.
-
1b1. App-Ointment prompts user that there are no patients in the current display.
-
2a. The index out of the bounds of the displayed list of patients.
-
2a1. App-Ointment warns user that the index is out of bounds and displays the bounds of the displayed list of patients.
Steps 2a1 is repeated until the index entered is correct/free from errors. Use case resumes from step 2.
-
2a1. App-Ointment warns user that the index is out of bounds and displays the bounds of the displayed list of patients.
UC07: Find patients by search fields
MSS
- User enters the
find-patient
command and corresponding subcommands. - App-Ointment changes the displayed list of patients to fit.
Extensions
-
1a. System detects an invalid subcommand format.
-
1a1. App-Ointment prompts user that syntax is incorrect and displays the expected format.
Steps 1a1 is repeated until the subcommand entered is correct/free from errors. Use case resumes from step 2.
-
1a1. App-Ointment prompts user that syntax is incorrect and displays the expected format.
-
2a. There are no doctors to display.
-
2a1. App-Ointment informs user that there are no patients to display.
Use case ends.
-
2a1. App-Ointment informs user that there are no patients to display.
UC08: List all patients
MSS
- User enters the
list-patient
command - App-Ointment displays all patients in the patient records.
UC09: Add a doctor
MSS
- User enters the
add-doctor
command and corresponding subcommands. - App-Ointment adds a new doctor to the doctor records.
Extensions
- Similar to
add-patient
command.
UC10: Clear Doctor Records
MSS
- User enters the
clear-doctor
command. - App-Ointment clears the patient records.
Use case ends.
Extensions
- Similar to
clear-patient
command.
UC11: Delete a doctor
MSS
- User enters the
delete-doctor
command and corresponding subcommands. - App-Ointment removes the patient from the doctor records.
Extensions
- Similar to
delete-patient
command.
UC12: Edit a doctor
MSS
- User enters the
edit-doctor
command and corresponding subcommands. - App-Ointment changes the details of the doctor.
Extensions
- Similar to
edit-patient
command.
UC13: Find doctors by search fields
MSS
- User enters the
find-doctor
command and corresponding subcommands. - App-Ointment changes the displayed list of doctors to fit.
Extensions
- Similar to
find-patient
command.
UC14: List all doctors
MSS
- User enters the
list-doctor
command - App-Ointment displays all doctors in the doctor records.
UC15: Add an appointment
MSS
- User enters the
add-appt
command and corresponding subcommands. - App-Ointment adds a new appointment to the appointment schedule.
Extensions
-
1a. App-Ointment detects an invalid subcommand format.
-
1a1. App-Ointment prompts user that syntax is incorrect and displays the expected format.
Steps 1a1 is repeated until the subcommand entered is correct/free from errors. Use case resumes from step 2.
-
1a1. App-Ointment prompts user that syntax is incorrect and displays the expected format.
-
1b. The patient index out of the bounds of the displayed list of patients.
-
1b1. App-Ointment warns user that the index is out of bounds and displays the bounds of the displayed list of patients.
Steps 1b1 is repeated until the index entered is correct/free from errors. Use case resumes from step 2.
-
1b1. App-Ointment warns user that the index is out of bounds and displays the bounds of the displayed list of patients.
-
1c. App-Ointment detects an existing appointment with the same patient or doctor at an overlapping appointment time.
-
1c1. App-Ointment warns user about the conflicting appointment.
-
1c2. App-Ointment suggest user to either change existing appointment details through an
edit-appt
command, before adding the new appointment again, or change the new appointment details.
Use case ends.
-
1c1. App-Ointment warns user about the conflicting appointment.
UC16: Clear Appointment Schedule
MSS
- User enters the
clear-patient
command. - App-Ointment clears the Appointment Schedule.
Use case ends.
UC17: Delete an appointment
MSS
- User enters the
delete-appt
command and corresponding subcommands. - App-Ointment removes the appointment from the appointment schedule.
Extensions
-
1a. App-Ointment detects an invalid subcommand format.
-
1a1. App-Ointment prompts user that syntax is incorrect and displays the expected format.
Steps 1a1 is repeated until the subcommand entered is correct/free from errors. Use case resumes from step 2.
-
1a1. App-Ointment prompts user that syntax is incorrect and displays the expected format.
-
1b. The currently displayed list of appointments is empty.
-
1b1. App-Ointment prompts user that there are no appointments in the current display.
Use case ends.
-
1b1. App-Ointment prompts user that there are no appointments in the current display.
-
2a. The index out of the bounds of the displayed list of appointments.
-
2a1. App-Ointment warns user that the index is out of bounds and displays the bounds of the displayed list of appointments.
Steps 2a1 is repeated until the index entered is correct/free from errors. Use case resumes from step 2.
-
2a1. App-Ointment warns user that the index is out of bounds and displays the bounds of the displayed list of appointments.
UC18: Edit an appointment
MSS
- User enters the
edit-appt
command and corresponding subcommands. - App-Ointment changes the details of the appointment.
Extensions
-
1a. App-Ointment detects an invalid subcommand format.
-
1a1. App-Ointment prompts user that syntax is incorrect and displays the expected format.
Steps 1a1 is repeated until the subcommand entered is correct/free from errors. Use case resumes from step 2.
-
1a1. App-Ointment prompts user that syntax is incorrect and displays the expected format.
-
1b. The currently displayed list of appointments is empty.
-
1b1. App-Ointment prompts user that there are no appointments in the current display.
Use case ends.
-
1b1. App-Ointment prompts user that there are no appointments in the current display.
-
2a. The index out of the bounds of the displayed list of appointments.
-
2a1. App-Ointment warns user that the index is out of bounds and displays the bounds of the displayed list of appointments.
Steps 2a1 is repeated until the index entered is correct/free from errors. Use case resumes from step 2.
-
2a1. App-Ointment warns user that the index is out of bounds and displays the bounds of the displayed list of appointments.
-
2b. App-Ointment detects an existing appointment having conflict with the new appointment.
-
2b1. App-Ointment warns user about the conflicting appointment.
-
2b2. App-Ointment suggest user to either change the other existing appointment details through a separate
edit-appt
command, before editing the current appointment again, or change the edit details of the current appointment.
Use case ends.
-
2b1. App-Ointment warns user about the conflicting appointment.
UC19: Find appointments by search fields
MSS
- User enters the
find-appt
command and corresponding subcommands. - App-Ointment changes the displayed list of appointments to fit.
Extensions
-
1a. System detects an invalid subcommand format.
-
1a1. App-Ointment prompts user that syntax is incorrect and displays the expected format.
Steps 1a1 is repeated until the subcommand entered is correct/free from errors. Use case resumes from step 2.
-
1a1. App-Ointment prompts user that syntax is incorrect and displays the expected format.
-
2a. There are no appointments to display.
-
2a1. App-Ointment informs user that there are no appointments to display.
Use case ends.
-
2a1. App-Ointment informs user that there are no appointments to display.
UC20: List all appointments
MSS
- User enters the
list-appt
command. - App-Ointment displays all appointments in the appointment schedule.
Non-Functional Requirements
Non-functional requirements specify the constraints under which App-Ointment is developed and operated.
Constraints:
- The system should be backward compatible with data produced by earlier versions of the system.
Technical requirements:
- Should work on any mainstream OS as long as it has Java
11
or above installed.
Performance requirements:
- Should be able to hold up to 1000 patients without a noticeable sluggishness in performance for typical usage.
- Should be able to hold up to 1000 doctors without a noticeable sluggishness in performance for typical usage.
- Should be able to hold up to 1000 appointments without a noticeable sluggishness in performance for typical usage.
Quality requirements:
- A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
Notes about project scope:
- The App-Ointment data file is private and local to the user.
Glossary
- Appointment Schedule: The list of appointments maintained in App-Ointment, arranged by appointment datetime.
- Command: An input containing the Command Word and Subcommands (if applicable).
-
Command Word: The word that defines the behaviour of the app: eg.
add-doctor
,list-appt
,delete-patient
, etc. - Doctor Records: The list of doctors maintained in App-Ointment.
- Mainstream OS: Windows, Linux, Unix, OS-X
- Patient Records: The list of patients maintained in App-Ointment.
- Person: Patient or Doctor
-
Subcommand: The prefixes and parameters that follow behind a Command Word entered by the user. eg.
n/Some Name
- System: The App-Ointment App
- User: The Receptionist, not the patient or doctor
Appendix: Instructions for manual testing
Given below are instructions to test the app manually.

Launch and shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Double-click the jar file Expected: Shows the GUI with a set of sample contacts. The window size may not be optimum.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
-
Adding a patient
-
Adding a new patient
-
Prerequisites: The patient to add must not already exist in the patient records.
-
Test case:
add-patient n/John Doe p/98765432 e/johnd@example.com a/John street, block 123, #01-01
Expected: The new patient is appended to the patient records, and the details of the patient are displayed in the status message. -
Test case:
add-patient n/John Doe e/johnd@example.com a/John street, block 123, #01-01
Expected: No patient is added. Error details are shown in the status message. -
Other incorrect
add-patient
commands to try:add-patient n/John Doe
,add-patient n/John Doe p/a2345678
,...
(where a field is missing, or the values are invalid)
-
-
Adding a duplicate patient
-
Prerequisites: The patient to add has the same
Name
as an existing patient in the patient records. -
Test case:
add-patient n/John Doe p/98765432 e/johnd@example.com a/John street, block 123, #01-01
Expected: No patient is added. Error details are shown in the status message. -
Missing fields and invalid value errors take precedence before duplicate patient errors.
-
Adding a doctor
-
Adding a new doctor
-
Prerequisites: The doctor to add must not already exist in the doctor records.
-
Test case:
add-doctor n/Dr Meredith Grey
Expected: The new doctor is appended to the doctor records, and the details of the doctor are displayed in the status message. -
Test case:
add-doctor n/
Expected: No doctor is added. Error details are shown in the status message. -
Other incorrect
add-doctor
commands to try:add-doctor n/Ca$h Money
,...
(where theName
value is invalid)
Expected: Similar to previous.
-
-
Adding a duplicate doctor
-
Prerequisites: The doctor to add has the same
Name
as an existing doctor in the doctor records. -
Test case:
add-doctor n/Dr Meredith Grey
Expected: No doctor is added. Error details are shown in the status message.
-
Adding an appointment
-
Adding a non-conflicting appointment while all patients and doctors are shown
-
Prerequisites: List all patients and doctors using the
list-patient
andlist-doctor
command.
There must be at least 1 patient and doctor in the patient records and doctor records respectively. -
Test case:
add-appt pt/1 dr/1 at/2021-01-01 00:00 to/2021-01-01 01:30
Expected: The appointment is appended to the appointment schedule, and the details of the appointment are displayed in the status message. -
Test case:
add-appt pt/1
Expected: No appointment is added. Error details are shown in the status message. -
Other incorrect
add-appt
commands to try:add-appt pt/0
,add-appt pt/1 dr/1 at/2021-01-01 dur/0H 0M
,...
(where a field is missing, values are invalid, or the indexes are out of bounds)
Expected: Similar to previous.
-
-
Adding a conflicting appointment
-
Prerequisites: The appointment to add is in conflict with an existing appointment in the appointment schedule.
There is an overlap in theTimeslot
fields with either the samePatient
orDoctor
. -
Test case:
add-appt pt/1 dr/1 at/2021-01-01 00:00 to/2021-01-01 01:30
Expected: No appointment is added. Error details are shown in the status message. -
Missing fields and invalid value errors take precedence before conflicting appointment errors.
-
Clearing patient records (or doctor records)
-
Clearing patient records while appointment schedule is not empty.
- Test case:
clear-patient
Expected: The patient records are not cleared. An error message will be returned.
- Test case:
-
Clearing patient records while appointment schedule is empty.
- Test case:
clear-patient
Expected: The patient records are cleared. It does not matter if there are entries in the patient records.
- Test case:
Note: This set of test cases can be similarly performed for doctor records by replacing mentions of clear-patient
with clear-doctor
.
Clearing appointment schedule
-
Clearing appointment schedule.
- Test case:
clear-appt
Expected: The appointment schedule is cleared. It does not matter if there are entries in the appointment schedule.
- Test case:
Deleting a patient (or doctor)
-
Deleting a patient while all patients with no existing appointments in the appointment schedule.
-
Prerequisites: List all persons using the
list-patient
command. Multiple persons in the list. -
Test case:
delete-patient 3
Expected: The third patient, Charlotte Olivero, is deleted from the patient records. Details of the deleted patient will be shown in the feedback message. -
Test case:
delete-patient 0
Expected: No patient is deleted. An error message will be returned. -
Test case:
delete-patient 1
Expected: An error message informing that the patient has existing appointments in the appointment schedule and a force delete command is required.
-
-
Force deleting a patient with existing appointments in the appointment schedule.
- Test case:
delete-patient --force 1
Expected: The first patient, Alex Karev, is deleted from the patient records, along with his existing appointments in the appointment schedule. Details of the deleted patient will be shown in the feedback message.
- Test case:
Note: This set of test cases can be similarly performed for doctors in the doctor records by replacing mentions of delete-patient
with delete-doctor
.
Editing a patient
-
Editing a patient
-
Prerequisites: The patient to edited must already exist in the patient records.
-
Test case:
edit-patient 1 p/98432567
Expected: The phone number of the first patient in the patient list is changed to 98432567, and the details of the patients are displayed in the status message. -
Test case:
edit-patient 2 e/
Expected: The email address of the second patient in the patient list is not changed. Error details are shown in the status message. Status bar remains the same. -
Other incorrect
edit-patient
commands to try:edit-patient 1 e/John*example.com
,...
(where theEmail
address is invalid)
Expected: Similar to previous.
-
-
Editing results in duplicated patients
-
Prerequisites: The edited name of the patient has the same
Name
as an existing patient in the patient records. -
Test case:
edit-patient 1 n/Dong
Expected: The name of patient 1 is not edited. Error details are shown in the status message. Status bar remains the same.
-
Editing a doctor
-
Editing a doctor
-
Prerequisites: The doctor to edited must already exist in the doctor records.
-
Test case:
edit-doctor 1 t/medicine
Expected: The tag of the first doctor in the doctor list is changed to medicine, and the details of the doctors are displayed in the status message. -
Test case:
edit-patient 2 t/
Expected: All the tags of the second doctor in the doctor list are cleared, and the details of the doctors are displayed in the status message. -
Other incorrect
edit-doctor
commands to try:edit-doctor 1
,...
(where no field to edit is provided)
Expected: An error message informing that at least one field to edit must be provided.
-
-
Editing results in duplicated doctors
-
Prerequisites: The edited name of the doctor has the same
Name
as an existing doctor in the doctor records. -
Test case:
edit-doctor 1 n/Dr Who
Expected: The name of doctor 1 is not edited. Error details are shown in the status message. Status bar remains the same.
-
Edit an appointment
-
Editing an appointment
-
Prerequisites: The appointment to edited must already exist in the appointment schedule.
-
Test case:
edit-appt 1 pt/2
Expected: The patient of the first appointment in the appointment schedule is changed to the second patient in the patient list, and the details of the appointments are displayed in the status message. -
Test case:
edit-appt 2 at/2021-05-08 09:00
Expected: The starting time of the second appointment in the appointment schedule is changed to 2021-05-08 09:00. If the starting time is the same or after the end time of the appointment, and error message will be shown indicating the timeslot end date and time must be strictly after the start date and time. -
Other incorrect
edit-appt
commands to try:edit-appt 1 at/2021-*-08 09:00
,...
(where the starting time format provided is invalid)
Expected: An error message informing ‘Invalid Date Time Format!’ is shown.
-
-
Editing results in conflicting appointments
- Prerequisites: The edited appointment has conflicting patient or doctor timeslots with an existing appointment in the appointment schedule.
- Test case:
edit-appt 1 to/2022-06-08 09:00
Expected: The end time of the first appointment in the appointment schedule is not changed. Error details are shown in the status message. Status bar remains the same.
Find patients by search fields
-
Finding a patient
-
Prerequisites: The patient to be found must exits in the patient list.
-
Test case:
find-patient John
Expected: The patient with name John is found and listed. -
Test case:
find-patient 84511556
Expected: 0 patient is found. This is becausefind-patient
command only search for keywords in the patient’s name field.
-
Note: This test case can be similarly performed for doctors in the doctor records by replacing find-patient
with find-doctor
.
Listing patient records (or doctor records, or appointment schedule)
-
Listing of all patients in the patient records when a predicate has been applied.
- Prerequisites: A
find-patient
command which filters the patient records must have been applied, and the original patient records must not have been empty. - Test case:
list-patient
Expected: All patients are listed, and applied predicates are removed. The resulting number of patients displayed will be more than what was previously displayed.
- Prerequisites: A
-
Listing of all patients in the patient records when no predicate has been applied.
- Test case:
list-patient
Expected: All patients are listed, and applied predicates are removed. The command will succeed, but since there were no predicates applied, there will be no visible changes to the patient records.
- Test case:
Note: This set of test cases can be similarly performed for doctors in the doctor records by replacing mentions of list-patient
with list-doctor
, and for appointments in the appointment schedule by replacing mentions of list-patient
with list-appt
.
Reading data from storage
-
Dealing with missing appointment schedule where patient and doctor records have been modified substantially.
-
Prerequisites: the patient and doctor records must have been modified from the sample patient and doctor records such that: there exists appointments in the sample appointment schedule which refer to a patient or doctor, whose UUID is not present in the patient or doctor records.
-
Test case: Delete the appointment schedule json file (default name
AppointmentSchedule.json
, in default locationdata/
)
Expected: App-Ointment will start with an empty appointment schedule.
-
-
Dealing with missing appointment schedule while patient and doctor records are the same as the sample patient and doctor records respectively.
- Test case: Delete the appointment schedule json file
Expected: App-Ointment will start with a sample Appointment Schedule.
- Test case: Delete the appointment schedule json file
-
Dealing with missing patient/doctor records
- Test case: Delete the patient records json file (default name
PatientRecords.json
, in default locationdata/
)
Expected: App-Ointment will start with an sample patient record. If the resultant sample patient record results in an appointment schedule with appointments which refer to a patient whose UUID is not present in the patient record, the appointment schedule will be treated as a corrupt data file.
- Test case: Delete the patient records json file (default name
Note: this set of test cases can be similarly performed for the doctor record json file by replacing mentions of PatientRecords.json
with DoctorRecords.json
.
-
Dealing with corrupted patient/doctor records
- Test case: edit
PatientRecords.json
such that there is now an invalid patient (e.g. editing the name of a patient such that it has a special character in it)
Expected: App-Ointment will start with an empty patient record and an empty appointment schedule.
- Test case: edit
Note: this set of test cases can be similarly performed for the doctor record json file by replacing mentions of PatientRecords.json
with DoctorRecords.json
.
-
Dealing with corrupted appointment schedule
- Test case: edit
AppointmentSchedule.json
such that there is now an invalid appointment (e.g. editing the uuid of a patient in the appointment schedule json such that the uuid does not belong to any patient in the patient records)
Expected: App-Ointment will start with an empty appointment schedule.
- Test case: edit
Appendix: Effort
- Built on AB-3’s Address Book, by creating an Appointment Management Software
- Extended and modified
Person
such thatPatient
andDoctor
classes inherit fromPerson
. - Created
Appointment
which takes in 2Person
s: aPatient
and aDoctor
, aTimeslot
, andTag
s. - Add, Clear, Edit, Find, List commands were added for all 3 categories of objects (
Patient
,Doctor
,Appointment
) (modified AB-3 Implementation forPatient
) - Added Up/Down Key Toggle Features for user to toggle between the next and previous commands they have input.
- Added prompting for the closest known command word to alert users in the event of a typographical error in the command word.
- Handled dependency issues regarding editing of patient/doctors.
- Met issues with replacement of person during an edit command,
- Implemented UUID field to act as a de-facto primary key for a
Person
, and use the UUIDs in anAppointment
instead of a direct reference to thePatient
andDoctor
objects. This is so that anAppointment
can uniquely identify aPatient
as well as aDoctor
, and still be able to listen to changes in a Patient. - Handled UI display of Patient, Doctor, Appointment to not display the UUID and to show the appropriate names instead, and performed the translation with high efficiency with Java Maps.
- Handled dependency issues regarding deleting of patient/doctors and clearing of patient/doctor records.
- Added exceptions when patient/ doctor has existing appointments in the appointment schedule
- Added force delete functionality for delete commands so that users can conveniently delete patients/ doctors with their associated appointments
- Modified
Model
,Storage
, andLogic
to be generic, to accept extensions of thePerson
class. -
Added Timeslot Parser, and related enums, so that user is able to be more flexible in entering date and time for their appointments.
- Added Tests regarding the new classes and commands